This code appears to be for a simple web-based image search engine. It consists of three main parts: an HTML file for the structure of the webpage, a CSS file for styling, and a JavaScript file for handling user interactions and making API requests. Let’s break down the code with explanations and comments:
Folder Structure
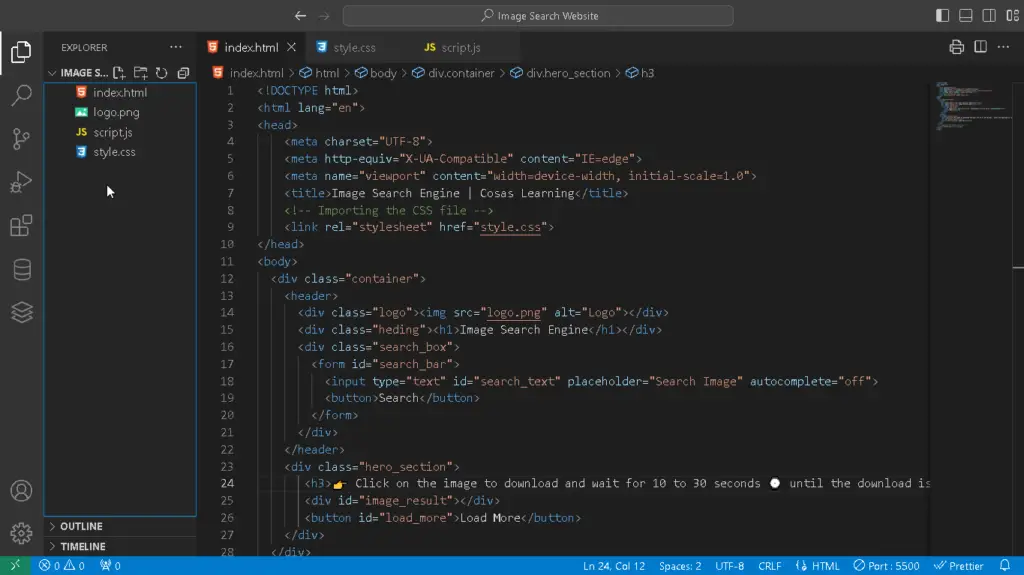
Resources
Prerequisite Sites
Image
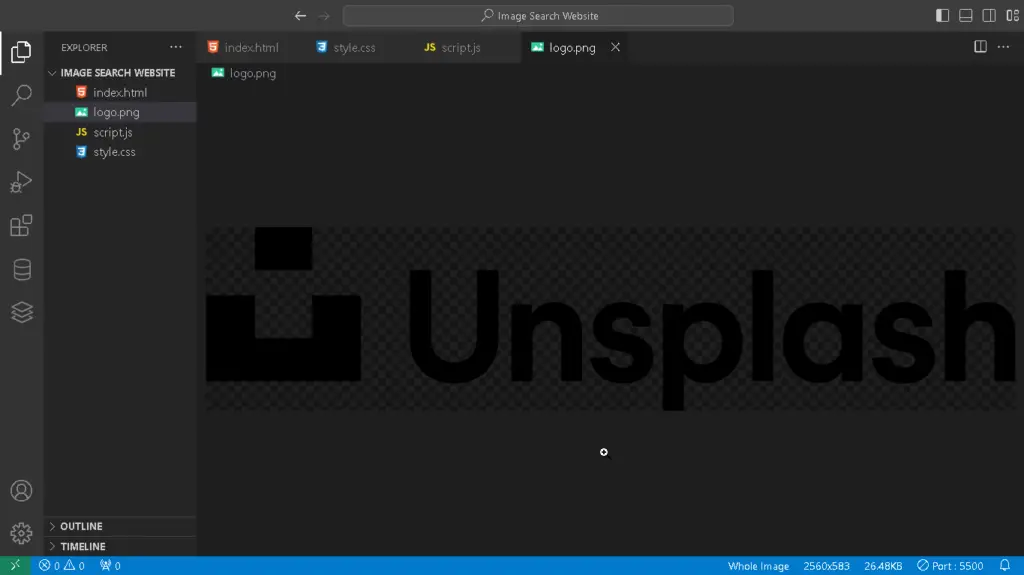
Codes
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Search Engine | Cosas Learning</title>
<!-- Importing the CSS file -->
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<header>
<div class="logo"><img src="logo.png" alt="Logo"></div>
<div class="heding"><h1>Image Search Engine</h1></div>
<div class="search_box">
<form id="search_bar">
<input type="text" id="search_text" placeholder="Search Image" autocomplete="off">
<button>Search</button>
</form>
</div>
</header>
<div class="hero_section">
<h3>👉 Click on the image to download and wait for 10 to 30 seconds ⌚ until the download is complete. ⏳</h3>
<div id="image_result"></div>
<button id="load_more">Load More</button>
</div>
</div>
<!-- Importing the JavaScript file -->
<script src="script.js"></script>
</body>
</html>
- This HTML file defines the structure of the webpage. It includes references to an external CSS file (
style.css
) for styling and an external JavaScript file (script.js
) for functionality. - It sets up a header with a logo, a title, and a search box for users to input their search queries.
- The
hero_section
contains a message, an image result container (image_result
), and a “Load More” button (load_more
).
CSS
/*----------------- IMPORT GOOGLE FONT -----------------*/
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600&display=swap');
/*----------------- BASE -----------------*/
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
/*----------------- VARIABLES -----------------*/
:root {
/* Colors */
--white_color : rgb(255, 255, 255);
--orange_color : rgb(246, 99, 53);
--black_color : rgb(0, 0, 0);
--background_color :linear-gradient(to top left, #b156d9, #213780);
--grey-color : rgb(228, 222, 222);
}
/*----------------- STYLING -----------------*/
.container {
width: 100%;
min-height: 100vh;
background-image: var(--background_color);
padding-bottom: 1rem;
}
header {
padding: 2rem 0;
display: flex;
align-items: center;
justify-content: space-around;
}
.logo {
width: 15rem;
height: 4rem;
}
.logo img {
width: 100%;
height: 100%;
}
h1 {
color: var(--white_color);
text-align: center;
padding: 1rem;
border-bottom: 0.2rem var(--orange_color) solid;
text-transform: uppercase;
}
.search_box {
width: 20rem;
height: 3rem;
}
form {
width: 100%;
height: 100%;
background-color:var(--grey-color);
display: flex;
align-items: center;
justify-content: center;
border-radius: 0.5rem;
}
form input {
height: 100%;
border: 0;
outline: 0;
background: transparent;
flex: 1;
color: var(--black_color);
font-size: 1rem;
padding: 0 0.8rem;
}
form input::placeholder {
color:var(--black_color);
}
form button {
padding: 0 1rem;
height: 100%;
background:var(--orange_color);
color:var(--white_color);
font-size: 1rem;
border: 0;
outline: 0;
border-top-right-radius: 0.5rem;
border-bottom-right-radius: 0.5rem;
cursor: pointer;
transition: 0.5s;
}
.hero_section {
display: flex;
flex-direction: column;
}
h3 {
color: var(--white_color);
padding: 2rem 0 2rem 4rem;
}
#load_more {
color: var(--white_color);
background: var(--orange_color);
font-size: 1rem;
padding: 1rem;
border: 0;
outline: 0;
border-radius: 0.5rem;
cursor: pointer;
margin: 0.6rem auto 8rem;
transition: 0.5s;
}
form button:hover, #load_more:hover {
color: var(--orange_color);
background: var(--white_color);
font-weight: bold;
}
#image_result {
width: 100%;
column-count: 3;
column-gap: 1.5rem;
padding: 2rem 4rem;
}
#image_result img {
width: 100%;
height: auto;
break-inside: avoid;
border-radius: 0.5rem;
}
- This CSS file defines the styling for the webpage elements. It includes rules for colors, fonts, layout, and various components of the page.
JavaScript
// Access key from Unsplash
const accesskey = "Here Enter Your Access Key";
// Variables
const searchbar = document.getElementById("search_bar");
const searchtext = document.getElementById("search_text");
const imageresult = document.getElementById("image_result");
const loadmore = document.getElementById("load_more");
// Using API
let keyword = "";
let page = 1;
async function searchImages(){
// Fetch images from Unsplash API based on user input
keyword = searchtext.value;
const url = `https:api.unsplash.com/search/photos?page=${page}&query=${keyword}&client_id=${accesskey}&per_page=15`;
const response = await fetch(url);
const data = await response.json();
if(page === 1){
imageresult.innerHTML = "";
}
const results = data.results;
results.map((result) => {
const image = document.createElement("img");
image.src = result.urls.small;
image.style.cursor = "pointer";
image.addEventListener("click", async () => {
try {
const response_img = await fetch(result.urls.full);
const file = await response_img.blob();
const link = document.createElement("a");
link.href = URL.createObjectURL(file);
link.download = 'Cosas_Learning_' + new Date().getTime();
link.click();
} catch {
alert("Failed to download the file!");
}
});
imageresult.appendChild(image);
});
}
loadmore.addEventListener("click", ()=> {
// Load more images when the "Load More" button is clicked
page++;
searchImages();
})
searchbar.addEventListener("submit", (e) => {
// Handle form submission when the user searches for images
e.preventDefault();
page = 1;
searchImages();
})
window.addEventListener("load", () => {
// Load random images when the page loads
searchtext.value = "Random Images";
searchImages();
});
- This JavaScript file handles the main functionality of the image search engine.
- It defines variables for various HTML elements and sets up event listeners for user interactions.
- The
searchImages
function fetches images from the Unsplash API based on user input, and it also handles the download functionality when an image is clicked. - Event listeners are set up to handle the “Load More” button click and form submission for searching images.
- When the page loads, it initially loads random images.
Conclusion
Overall, this code combines HTML, CSS, and JavaScript to create a simple image search engine that allows users to search for images and download them. It uses the Unsplash API to fetch and display images based on user queries.
YouTube Video
Download Source Code
Don’t forget to share this post!
Click Here : To Show Your Support! 😍