This project is a simple AI Image Generator web application that allows users to generate AI images based on a provided text prompt. It leverages the OpenAI API to create images and offers the option to download them. The project consists of HTML, CSS, and JavaScript code. Below, we have provided an optimized version of the code with comments to explain each step.
Folder Structure
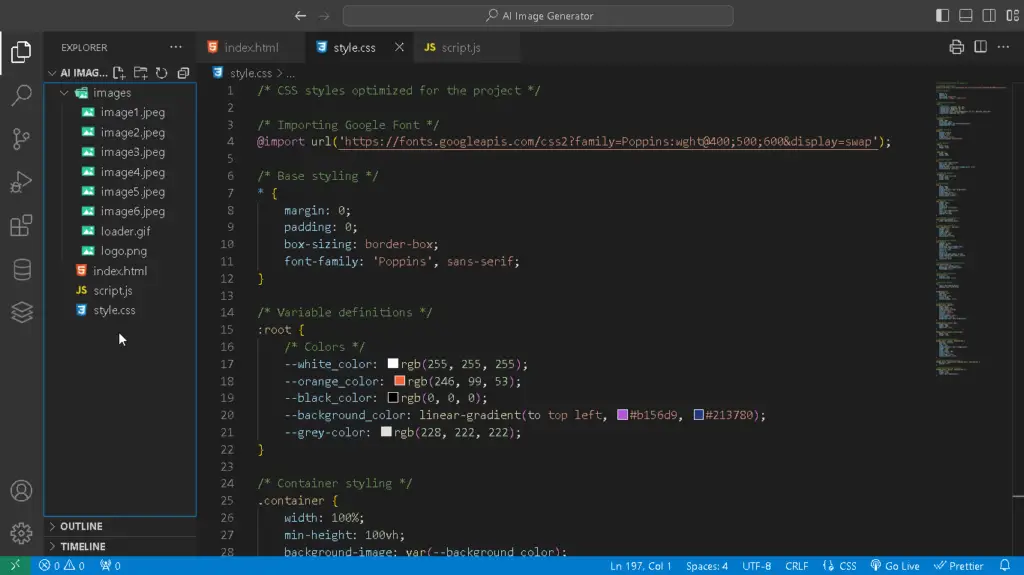
Resources
Prerequisite Sites
Images
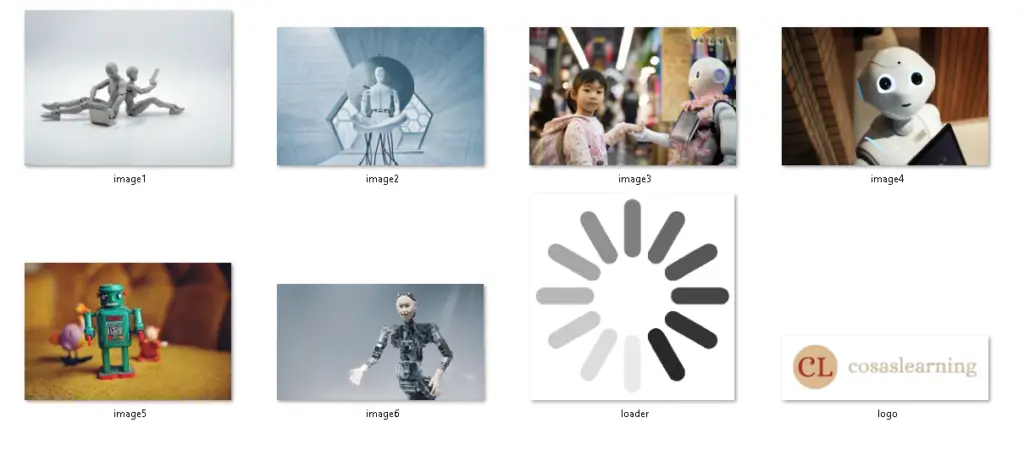
Codes
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Search Engine | Cosas Learning</title>
<!-- Importing the CSS file -->
<link rel="stylesheet" href="style.css">
<!-- CDN Font Awesome -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.2/css/all.min.css"
integrity="sha512-z3gLpd7yknf1YoNbCzqRKc4qyor8gaKU1qmn+CShxbuBusANI9QpRohGBreCFkKxLhei6S9CQXFEbbKuqLg0DA=="
crossorigin="anonymous" referrerpolicy="no-referrer" />
</head>
<body>
<div class="container">
<header>
<div class="logo"><img src="images/logo.png" alt="Logo"></div>
<div class="heding"><h1>AI Image Generator</h1></div>
</header>
<h3>👉 Convert Your Text Into An AI-Generated Images And Also Download Them 😊</h3>
<div class="prompt_box">
<form action="#" id="prompt_bar">
<input type="text" id="prompt_text" placeholder="Provide a prompt for your image" autocomplete="off" required>
<select class="img_quantity">
<!-- Options for selecting the number of images to generate -->
<!-- Default selection: 3 images -->
<option value="1">1 Image</option>
<option value="2">2 Images</option>
<option value="3" selected>3 Images</option>
<option value="4">4 Images</option>
<option value="5">5 Images</option>
<option value="6">6 Images</option>
<option value="7">7 Images</option>
<option value="8">8 Images</option>
<option value="9">9 Images</option>
<option value="10">10 Images</option>
</select>
<select class="img_size">
<!-- Options for selecting the image size -->
<!-- Default selection: 512x512 pixels -->
<option value="256x256">Size: 256x256</option>
<option value="512x512" selected>Size: 512x512</option>
<option value="1024x1024">Size: 1024x1024</option>
</select>
<button>Generate</button>
</form>
</div>
<div class="hero_section">
<div id="image_result">
<!-- Generated images will be displayed here -->
<div class="img_box">
<img src="images/image1.jpeg">
</div>
<div class="img_box">
<img src="images/image2.jpeg">
</div>
<div class="img_box">
<img src="images/image3.jpeg">
</div>
<div class="img_box">
<img src="images/image4.jpeg">
</div>
<div class="img_box">
<img src="images/image5.jpeg">
</div>
<div class="img_box">
<img src="images/image6.jpeg">
</div>
</div>
</div>
</div>
<!-- Importing the JavaScript file -->
<script src="script.js"></script>
</body>
</html>
- The HTML file sets up the structure of the web page for the AI image generator.
- It includes references to an external CSS file (
style.css
) for styling and an external JavaScript file (script.js
) for functionality. - The page contains a header with a logo and title, a form for user input, and a section for displaying generated images.
CSS
/* CSS styles optimized for the project */
/* Importing Google Font */
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600&display=swap');
/* Base styling */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
/* Variable definitions */
:root {
/* Colors */
--white_color: rgb(255, 255, 255);
--orange_color: rgb(246, 99, 53);
--black_color: rgb(0, 0, 0);
--background_color: linear-gradient(to top left, #b156d9, #213780);
--grey-color: rgb(228, 222, 222);
}
/* Container styling */
.container {
width: 100%;
min-height: 100vh;
background-image: var(--background_color);
padding-bottom: 1rem;
}
/* Header styling */
header {
padding: 2rem 0;
margin: 0 3rem;
display: flex;
align-items: center;
justify-content: space-between;
}
/* Logo styling */
.logo {
width: 20rem;
height: 6rem;
}
.logo img {
width: 100%;
height: 100%;
}
/* Heading styling */
h1 {
color: var(--white_color);
text-align: center;
padding: 1rem;
border-bottom: 0.2rem var(--orange_color) solid;
text-transform: uppercase;
}
/* Prompt box styling */
.prompt_box {
margin: 1rem auto 2rem;
width: 50rem;
height: 3rem;
}
/* Form styling */
form {
width: 100%;
height: 100%;
background-color: var(--grey-color);
display: flex;
align-items: center;
justify-content: center;
border-radius: 0.5rem;
}
/* Input field styling */
form input {
height: 100%;
border: 0;
outline: 0;
background: transparent;
flex: 1;
color: var(--black_color);
font-size: 1rem;
padding: 0 0.8rem;
}
/* Placeholder text styling */
form input::placeholder {
color: var(--black_color);
}
/* Select boxes styling */
form .img_quantity, .img_size {
outline: none;
border: none;
height: 44px;
background: none;
font-size: 1rem;
margin-right: 0.5rem;
}
/* Generate button styling */
form button {
padding: 0 1rem;
height: 100%;
background: var(--orange_color);
color: var(--white_color);
font-size: 1rem;
border: 0;
outline: 0;
border-top-right-radius: 0.5rem;
border-bottom-right-radius: 0.5rem;
cursor: pointer;
transition: 0.5s;
}
/* Hover effect for Generate button */
form button:hover {
color: var(--orange_color);
background: var(--white_color);
font-weight: bold;
}
/* Hero section styling */
.hero_section {
display: flex;
flex-direction: column;
}
/* Additional styling */
h3 {
color: var(--white_color);
padding: 2rem 0 2rem 4rem;
}
#image_result {
display: flex;
gap: 1rem;
flex-wrap: wrap;
margin: 3rem;
justify-content: center;
}
/* Image box styling */
#image_result .img_box {
width: 25rem;
aspect-ratio: 1/1;
border-radius: 0.5rem;
overflow: hidden;
position: relative;
display: flex;
justify-content: center;
align-items: center;
background-color: var(--grey-color);
}
#image_result .img_box img {
width: 100%;
height: 100%;
object-fit: cover;
}
#image_result .img_box.loading img {
width: 5rem;
height: 5rem;
}
/* Download button styling */
#image_result .img_box .download-btn {
position: absolute;
top: 1rem;
right: 1rem;
background-color: var(--orange_color);
height: 2rem;
width: 2rem;
border-radius: 50%;
display: none;
align-items: center;
justify-content: center;
}
/* Show download button on hover */
#image_result .img_box:not(.loading):hover .download-btn {
display: flex;
}
/* Download button icon styling */
#image_result .img_box .download-btn i {
width: 1rem;
height: 1rem;
color: var(--white_color);
}
- The CSS file defines styles for various elements on the page, including colors, fonts, layout, and components such as headers, forms, and buttons.
JavaScript
// Variables
const promptBar = document.querySelector("#prompt_bar");
const imageresult = document.querySelector("#image_result");
const downloadBtn = document.querySelector(".download-btn");
// Replace 'your-api-key' with your actual OpenAI API key
const openaiAPIKey = "your-api-key";
let isImgGen = false;
// Function to update image boxes with generated images
const updateImgBoxes = (imgBoxArray) => {
imgBoxArray.forEach((imgObject, index) => {
const imgBox = imageresult.querySelectorAll(".img_box")[index];
const imgElement = imgBox.querySelector("img");
const downloadBtn = imgBox.querySelector(".download-btn");
const aiImgGenerate = `data:image/jpeg;base64,${imgObject.b64_json}`;
imgElement.src = aiImgGenerate;
imgElement.onload = () => {
imgBox.classList.remove("loading");
downloadBtn.setAttribute("href", aiImgGenerate);
downloadBtn.setAttribute("download", `Cosas_Learning_${new Date().getTime()}.jpg`);
}
})
}
// Function to generate AI images
const generateAIImages = async (userPrompt, imgQuntity, imgSize) => {
try {
const response = await fetch('https://api.openai.com/v1/images/generations', {
method : "POST",
headers : {
"Content-Type": "application/json",
"Authorization": `Bearer ${openaiAPIKey}`
},
body:JSON.stringify({
prompt : userPrompt,
n: parseInt(imgQuntity),
size : imgSize,
response_format :"b64_json"
})
});
if(!response.ok) throw new Error("Failed to generate AI Images! Please try again.")
const { data } = await response.json();
console.log(data);
updateImgBoxes([...data]);
} catch (error) {
alert(error.message);
imageresult.style.display = "none";
} finally {
isImgGen = false;
}
}
// Function to handle the form submission
const handlePrompt = (e) => {
e.preventDefault();
if(isImgGen) return;
isImgGen = true;
imageresult.style.display = "flex";
const userPrompt = e.srcElement[0].value;
const imgQuntity = e.srcElement[1].value;
const imgSize = e.srcElement[2].value;
// Create loading placeholders for images
const imgBoxes = Array.from({length: imgQuntity}, () =>
`<div class="img_box loading">
<img src="images/loader.gif">
<a href="#" class="download-btn">
<i class="fa-solid fa-download"></i>
</a>
</div>`
).join("");
imageresult.innerHTML = imgBoxes;
generateAIImages(userPrompt, imgQuntity, imgSize);
}
// Add event listener for form submission
promptBar.addEventListener("submit", handlePrompt);
- The JavaScript file provides the main functionality of the AI image generator.
- It defines variables to select HTML elements and functions to update image boxes and generate AI images using the OpenAI API.
- Event listeners are set up to handle form submission and user interactions.
This web application provides a user-friendly interface for generating AI images based on user prompts. It leverages the OpenAI API and allows users to select the number and size of images to generate. The generated images are displayed with the option to download them. The code has been optimized and well-commented for clarity and functionality. Feel free to customize it further to meet your specific requirements or integrate it into a larger AI Image Generator project.
YouTube Video
Download Source Code
Don’t forget to share this post!
Click Here : To Show Your Support! 😍