Source Code Of Gradient Generator Using HTML, CSS And JavaScript. Learn How To Make Gradient Generator And Get Free Source Code.
Folder Structure
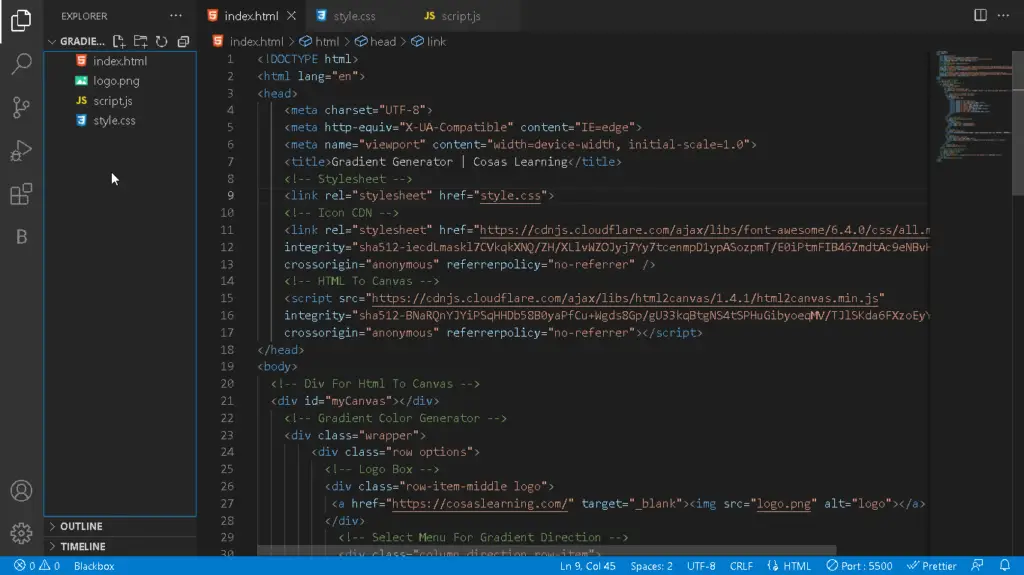
Resources
Prerequisite Sites
- https://fontawesome.com/
- https://fonts.google.com/
- https://cdnjs.cloudflare.com/ajax/libs/html2canvas/1.4.1/html2canvas.min.js
Image
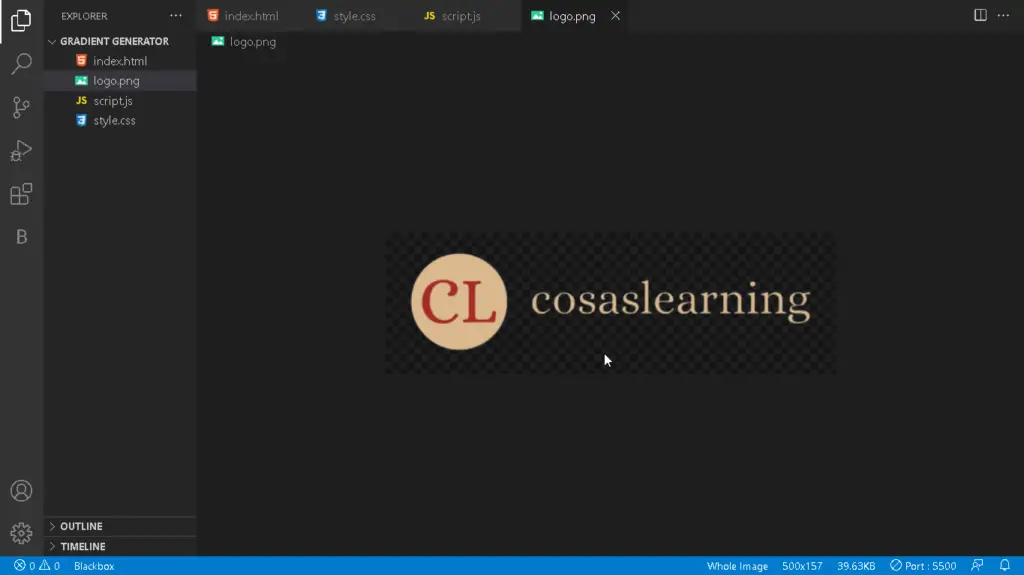
Codes
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Gradient Generator | Cosas Learning</title>
<!-- Stylesheet -->
<link rel="stylesheet" href="style.css">
<!-- Icon CDN -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.0/css/all.min.css"
integrity="sha512-iecdLmaskl7CVkqkXNQ/ZH/XLlvWZOJyj7Yy7tcenmpD1ypASozpmT/E0iPtmFIB46ZmdtAc9eNBvH0H/ZpiBw=="
crossorigin="anonymous" referrerpolicy="no-referrer" />
<!-- HTML To Canvas -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/html2canvas/1.4.1/html2canvas.min.js"
integrity="sha512-BNaRQnYJYiPSqHHDb58B0yaPfCu+Wgds8Gp/gU33kqBtgNS4tSPHuGibyoeqMV/TJlSKda6FXzoEyYGjTe+vXA=="
crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<!-- Div For Html To Canvas -->
<div id="myCanvas"></div>
<!-- Gradient Color Generator -->
<div class="wrapper">
<div class="row options">
<!-- Logo Box -->
<div class="row-item-middle logo">
<a href="https://cosaslearning.com/" target="_blank"><img src="logo.png" alt="Cosas Learning Logo"></a>
</div>
<!-- Select Menu For Gradient Direction -->
<div class="column direction row-item">
<p>Direction</p>
<div class="select-box">
<select>
<option value="to top">Top</option>
<option value="to top right">Top right</option>
<option value="to right">Right</option>
<option value="to bottom right">Bottom right</option>
<option value="to bottom">Bottom</option>
<option value="to bottom left">Bottom Left</option>
<option value="to left">Left</option>
<option value="to top left" selected>Top left</option>
</select>
</div>
</div>
<!-- Color Input Box -->
<div class="column palette row-item">
<p>Colors</p>
<div class="colors">
<input type="color" value="#8b42cf">
<input type="color" value="#7bda23">
</div>
</div>
<!-- Textarea -->
<div class="row-item-big">
<textarea disabled>background-image: linear-gradient(to left top, #977DFE, #6878FF);</textarea>
</div>
<!-- Buttons -->
<div class="buttons row-item">
<button class="copy">Copy Code</button>
<button class="download tooltip"><i class="fa-solid fa-download"></i></button>
</div>
</div>
<!-- Gradient Box -->
<div class="gradient-box"></div>
</div>
<!-- End Gradient Color Generator -->
<!-- Ramdom Gradient Colors -->
<ul class="container"></ul>
<!-- Refresh Button -->
<button class="refresh"><i class="fa-solid fa-arrows-rotate"></i> Refresh</button>
<!-- Script -->
<script src="script.js"></script>
</body>
</html>
CSS
/*----------------- IMPORT GOOGLE FONT -----------------*/
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600&display=swap');
/*----------------- BASE -----------------*/
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
/*----------------- VARIABLES -----------------*/
:root {
/* Colors */
--white_color : rgb(255, 255, 255);
--orange_color : rgb(246, 99, 53);
--black_color : rgb(0, 0, 0);
--background_color : rgb(27, 31, 41);
--grey-color : rgb(128, 128, 128);
--hover-color : rgb(247, 121, 2);
}
/*----------------- STYLING -----------------*/
body {
background: var(--background_color);
}
#myCanvas {
background-color:var(--orange_color);
position: absolute;
width: 64rem;
height: 44rem;
top: -150%;
}
.wrapper {
width: 100%;
height: 100%;
box-shadow: 0 0.9375rem 1.875rem rgba(0,0,0,0.06);
}
.wrapper .row {
width: 100%;
height: 8rem;
display: flex;
justify-content: center;
align-items: center;
gap: 1rem;
flex-wrap: wrap;
padding: 0 1.5rem;
color: var(--white_color);
}
.row-item {
flex: 0.5;
}
.row-item-middle {
flex: 0.7;
}
.row-item-big {
flex: 1;
display: flex;
align-items: center;
}
.logo {
width: 100%;
height: 100%;
display: flex;
align-items: center;
}
.logo img {
width: 100%;
height: 100%;
}
.wrapper .gradient-box {
width: 100%;
height: 30rem;
background: linear-gradient(to left top, #8b42cf, #7bda23);
}
.options p {
font-size: 1.1rem;
margin-bottom: 0.5rem;
}
.row :where(.column) {
width: calc(100% / 2 - 0.75rem);
}
.options .select-box {
border-radius: 0.3125rem;
padding: 0.625rem 0.9375rem;
border: 0.125rem solid var(--orange_color);
}
.select-box select {
cursor: pointer;
color: var(--white_color);
border: none;
outline: none;
font-size: 1.12rem;
background: var(--background_color);
}
.palette input {
height: 2.5rem;
cursor: pointer;
width: calc(100% / 2 - 0.3125rem);
}
.wrapper textarea {
color: var(--white_color);
font-size: 1.05rem;
resize: none;
width: 100%;
padding: 0.625rem 0.9375rem;
border-radius: 0.3125rem;
border: 0.125rem solid var(--orange_color);
}
.buttons {
display: flex;
align-items: center;
justify-content: center;
}
.buttons .copy, .download {
padding :0.9375rem;
border: none;
outline: none;
color:var(--white_color);
background: var(--orange_color);
border: 0.125rem solid var(--white_color);
font-size: 1.09rem;
border-radius: 0.3125rem;
cursor: pointer;
transition: 0.1s ease;
}
.buttons .download {
margin-left: 0.5rem;
}
.buttons .copy:hover, .download:hover {
background: var(--hover-color);
}
.container {
margin: 1.25rem;
display: flex;
justify-content: space-around;
flex-wrap: wrap;
}
.container .color {
margin: 0.75rem;
padding: 0.4375rem;
list-style: none;
text-align: center;
background: var(--white_color);
border-radius: 0.375rem;
box-shadow: 0 0.625rem 1.5625rem rgba(52,87,220,0.08);
transition: all 0.3s ease;
}
.container .color:active {
transform: scale(0.95);
}
.color .rect-box {
width: 11.5625rem;
height: 11.75rem;
border-radius: 0.25rem;
position: relative;
}
.ranDownload {
position: absolute;
top: 1rem;
right: 1rem;
font-size: 1.5rem;
cursor: pointer;
}
.ranDownload:hover {
color: var(--white_color);
}
.tooltip {
position: relative;
}
.tooltip:hover::before {
content: "Download .jpg";
position: absolute;
top: 125%;
left: 50%;
transform: translateX(-50%);
padding: 0.4rem;
border-radius: 0.3125rem;
background-color: #333;
font-weight: 700;
color: var(--white_color);
font-size: 0.875rem;
}
.color:hover .rect-box {
filter: brightness(107%);
}
.color .hex-value {
display: block;
cursor: pointer;
color: var(--black_color);
user-select: none;
font-weight: 500;
font-size: 1.15rem;
margin: 0.75rem 0 0.5rem;
text-transform: uppercase;
transition: all 0.1s;
}
.color .hex-value:hover {
color: var(--orange_color);
font-weight: 700;
}
.refresh {
position: fixed;
right: 2%;
bottom: 1.875rem;
color: var(--white_color);
cursor: pointer;
outline: none;
font-weight: 500;
font-size: 1.1rem;
border-radius: 0.3125rem;
background:var(--orange_color);
padding: 0.8125rem 1.25rem;
border: 0.125rem solid var(--white_color);
box-shadow: 0 0.9375rem 1.875rem rgba(52,87,220,0.2);
transition: all 0.3s ease;
}
.refresh:hover {
background: var(--hover-color);
}
/*----------------- MEDIA QUERIES -----------------*/
@media screen and (max-width: 1024px) {
.row-item-big {
display: none;
}
}
@media screen and (max-width: 768px) {
.logo {
margin-top: 1rem;
}
.logo img{
width: 25rem !important;
height: 100%;
}
.row-item {
width: 70%;
}
.row-item-big {
display: flex;
width: 70%;
}
.wrapper .row {
height: auto;
flex-direction: column;
padding: 0 0 2rem 0;
gap: 3rem;
}
.logo {
width: 100%;
height: 50%;
display: flex;
align-items: center;
justify-content: center;
}
.logo img {
width: 100%;
height: 100%;
}
.refresh {
font-size: 1rem;
}
}
@media screen and (max-width: 425px) {
.logo img {
width: 18.75rem !important;
height: 100%;
}
.refresh {
font-size: 0.8rem;
padding: 0.625rem 0.625rem;
border: 0.0625rem solid var(--white_color);
}
.wrapper .row {
gap: 2rem;
}
.options .colors {
display: flex;
justify-content: space-between;
}
}
@media screen and (max-width: 320px) {
.logo img {
width: 14.0625rem !important;
height: 100%;
}
.wrapper .row {
gap: 1.5rem;
}
.row-item {
width: 80%;
}
.row-item-big {
width: 80%;
}
}
JavaScript
//----- Gradient Color Variables -----//
const gradientBox = document.querySelector(".gradient-box");
const selectMenu = document.querySelector(".select-box select");
const colorInputs = document.querySelectorAll(".colors input");
const textarea = document.querySelector("textarea");
const refreshBtn = document.querySelector(".refresh");
const copyBtn = document.querySelector(".copy");
const download = document.querySelector(".download");
const ranDownload = document.querySelector(".ranDownload");
const rowItemBig = document.querySelector(".row-item-big");
//----- Generating a random color in hexadecimal format. Example: #5665E9 -----//
const getRandomColor = () => {
const randomHex = Math.floor(Math.random() * 0xffffff).toString(16);
return `#${randomHex.padStart(6, "0")}`;
}
//----- Generating Gradient Color -----//
const generateGradient = (isRandom) => {
if(isRandom) { // If isRandom is true, update the colors inputs value with random colors
colorInputs[0].value = getRandomColor();
colorInputs[1].value = getRandomColor();
}
// Creating a gradient string using the select menu value with color input values
const gradient = `linear-gradient(${selectMenu.value}, ${colorInputs[0].value}, ${colorInputs[1].value})`;
gradientBox.style.background = gradient;
textarea.value = `background: ${gradient};`;
}
//----- Header's Copy Button -----//
const copyCode = () => {
// Copying textarea value and updating the copy button text
navigator.clipboard.writeText(textarea.value);
copyBtn.innerHTML = "Copied ✔ ";
setTimeout(() => copyBtn.innerText = "Copy Code", 1000);
}
copyBtn.addEventListener("click", copyCode);
//----- When click on textarea it'll copy textarea value -----//
rowItemBig.addEventListener("click", copyCode);
//----- Getting Values For Download Gradient Image -----//
download.addEventListener("click", () => downloadGradient(selectMenu.value, colorInputs[0].value, colorInputs[1].value));
//----- Random Gradient Colors Vars -----//
const container = document.querySelector(".container");
const refreshBtn2 = document.querySelector(".refresh-btn");
const maxPaletteBoxes = 30;
//----- Random Gradient Colors -----//
const generatePalette = () => {
container.innerHTML = ""; // clearing the container
for (let i = 0; i < maxPaletteBoxes; i++) {
let colorRanOne = getRandomColor();
let colorRanTwo = getRandomColor();
// creating a new 'li' element and inserting it to the container
const color = document.createElement("li");
color.classList.add("color");
color.innerHTML = `<div class="rect-box" style="background:linear-gradient(${selectMenu.value}, ${colorRanOne}, ${colorRanTwo});">
<span class="ranDownload" title="Download .jpg"><i class="fa-solid fa-download"></i></span></div>
<span class="hex-value">Copy Code</span>`;
// adding click events to current li element to copy the color and download image
const copyText = color.querySelector(".hex-value");
const ranDownload = color.querySelector(".ranDownload");
copyText.addEventListener("click", () => copyColor(color, selectMenu.value, colorRanOne, colorRanTwo));
ranDownload.addEventListener("click", () => downloadGradient(selectMenu.value, colorRanOne, colorRanTwo));
container.appendChild(color);
}
}
generatePalette();
//----- Random Gradient Color Copy Button -----//
const copyColor = (elem, selectDirection, firstColor, secondColor) => {
const colorElement = elem.querySelector(".hex-value");
// Copying the hex value, updating the text to copied,
// and changing text back to original hex value after 1 second
const codeText = `background:linear-gradient(${selectDirection}, ${firstColor}, ${secondColor});`
navigator.clipboard.writeText(codeText).then(() => {
colorElement.innerHTML = "Copied ✔ ";
setTimeout(() => colorElement.innerText = "Copy Code", 1000);
}).catch(() => alert("Failed to copy the color code!")); // showing alert if color can't be copied
}
//----- Calling generateGradient & generatePalette function on each color input clicks -----//
colorInputs.forEach(input => {
input.addEventListener("input", () => {
generateGradient(false);
generatePalette();
})
});
//----- Calling generateGradient function on change selectMenu values & also changing random gradient colors direction according to selectMenu -----//
selectMenu.addEventListener("change", () => {
generateGradient(false);
// Get all the div elements within li elements
const clrdirections = document.querySelectorAll("li > div");
// Loop through each element and update their background gradient direction
clrdirections.forEach((clrdirection) => {
const currentBgImageStyle = window.getComputedStyle(clrdirection).getPropertyValue("background-image");
const currentGradientDirection = currentBgImageStyle.match(/to\s+(top|bottom|left|right)(?:\s+(top|bottom|left|right))?/i);
if (currentGradientDirection !== null) {
clrdirection.style.backgroundImage = currentBgImageStyle.replace(currentGradientDirection[0], selectMenu.value);
} else {
var backgroundColor = clrdirection.style.backgroundImage;
// Extract the two colors
var colors = backgroundColor.match(/rgba?\([^)]+\)|[a-f\d]{3,6}/ig);
// Store the first and second colors in separate variables
var firstColor = colors[0];
var secondColor = colors[1];
clrdirection.style.backgroundImage = `linear-gradient(${selectMenu.value}, ${firstColor}, ${secondColor}`;
}
});
});
//----- Refresh Button -----//
refreshBtn.addEventListener("click", () => {
generateGradient(true);
generatePalette();
});
//----- Download Image ( html to canvas) -----//
function downloadGradient(directionDown, firstclrDown, secondclrDown) {
const canvasDiv = document.getElementById("myCanvas");
const ranBackDown = `linear-gradient(${directionDown}, ${firstclrDown}, ${secondclrDown})`;
canvasDiv.style.backgroundImage = ranBackDown;
// Capture the HTML element as an image using html2canvas
html2canvas(canvasDiv).then(function(canvas) {
// Convert the canvas to a data URL and download as a JPG file
var link = document.createElement("a");
link.download = "Gradient-Cosas-Learning.jpg";
link.href = canvas.toDataURL("image/jpeg");
link.click();
});
}
YouTube Video
Download Source Code
Don’t forget to share this post!
Click Here : To Show Your Support! 😍