This project is a web-based Text To Speech converter that allows users to enter text and convert it into spoken words. The code is implemented using HTML, CSS, and JavaScript, and it provides a user-friendly interface for text input and voice selection. Below, we will explain the code structure and functionality.
Folder Structure
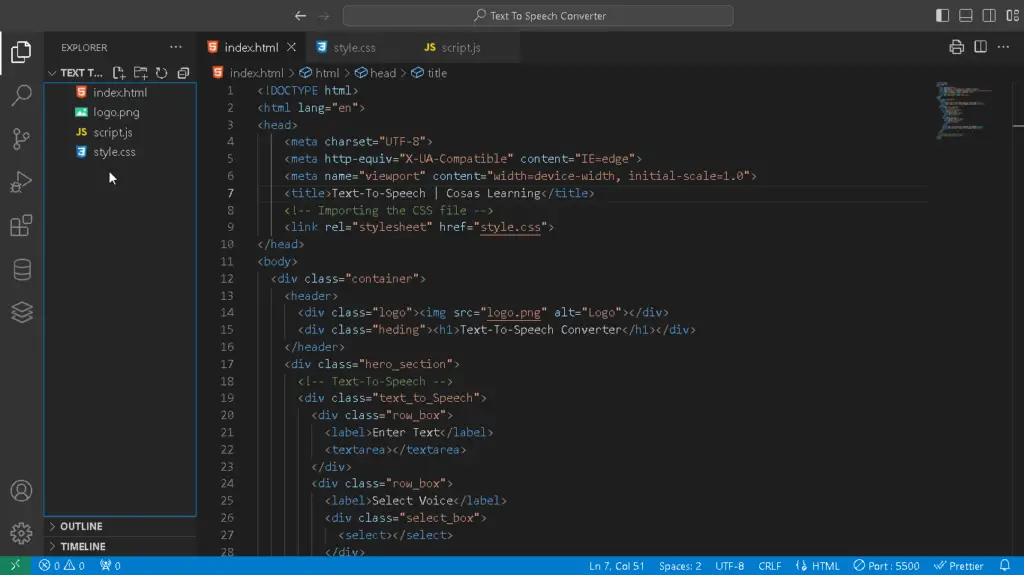
Resources
Prerequisite Sites
Images
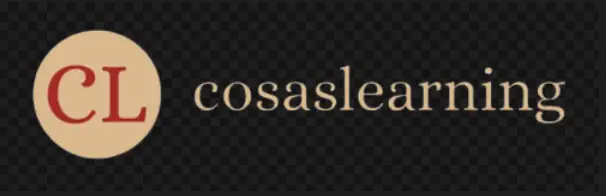
Codes
HTML
index.html<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Text-To-Speech | Cosas Learning</title> <!-- Importing the CSS file --> <link rel="stylesheet" href="style.css"> </head> <body> <div class="container"> <header> <div class="logo"><img src="logo.png" alt="Logo"></div> <div class="heding"><h1>Text-To-Speech Converter</h1></div> </header> <div class="hero_section"> <!-- Text-To-Speech --> <div class="text_to_Speech"> <div class="row_box"> <label>Enter Text</label> <textarea></textarea> </div> <div class="row_box"> <label>Select Voice</label> <div class="select_box"> <select></select> </div> </div> <button id="convert_speech">Convert To Speech</button> <button id="clearBtn" class="hide">Clear Text</button> </div> </div> </div> <!-- Importing the JavaScript file --> <script src="script.js"></script> </body> </html>
- The HTML file sets up the structure of the web page for a Text To Speech (TTS) converter.
- It includes references to external resources like the Font Awesome icon library and external CSS and JavaScript files.
- The page includes a container, header, and a section for text input, voice selection, and conversion buttons.
CSS
style.css/* CSS styles optimized for the project */ /* Importing Google Font */ @import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600&display=swap'); /* Base styling */ * { margin: 0; padding: 0; box-sizing: border-box; font-family: 'Poppins', sans-serif; } /* Variable definitions */ :root { /* Colors */ --white_color: rgb(255, 255, 255); --orange_color: rgb(255, 165, 89); --orange_dark_color: rgb(255, 96, 0); --background_color: linear-gradient(to top left, #b156d9, #213780); --box_shadow : rgba(0,0,0,0.1); } /* Container styling */ .container { width: 100%; min-height: 100vh; background-image: var(--background_color); padding-bottom: 1rem; } /* Header styling */ header { padding: 2rem 0; margin: 0 3rem; display: flex; align-items: center; justify-content: space-between; } /* Logo styling */ .logo { width: 20rem; height: 6rem; } .logo img { width: 100%; height: 100%; } /* Heading styling */ h1 { color: var(--white_color); text-align: center; padding: 1rem; border-bottom: 0.2rem var(--orange_color) solid; text-transform: uppercase; } /* Hero Section*/ .hero_section { display: flex; justify-content: center; align-items: center; flex-direction: column; } /* Text-To-Speech Styling Start*/ .text_to_Speech { width: 23.125rem; margin-top: 3rem; } .row_box { display: flex; margin-bottom: 1.25rem; flex-direction: column; } .row_box label { font-size: 1.2rem; font-weight: bold; color: var(--white_color); margin-bottom: 0.313rem; } .text_to_Speech :where(textarea, select, button) { outline: none; width: 100%; height: 100%; border: none; border-radius: 0.313rem; } .row_box textarea { resize: none; height: 6.875rem; font-size: 1rem; padding: 0.5rem 0.625rem; border: 0.125rem solid var(--orange_color); box-shadow: 0rem 0rem 0.625rem 1rem var(--box_shadow); } .row_box textarea::-webkit-scrollbar { width: 0rem; } .row_box .select_box { height: 2.938rem; display: flex; padding: 0 0.625rem; align-items: center; border-radius: 0.313rem; justify-content: center; border: 0.125rem solid var(--orange_color); box-shadow: 0rem 0rem 0.625rem 1rem var(--box_shadow); } .row_box select { font-size: 1rem; background: none; cursor: pointer; } .row_box select::-webkit-scrollbar { width: 0.5rem; } .row_box select::-webkit-scrollbar-track { background: var(--white_color); } .row_box select::-webkit-scrollbar-thumb { background: var(--orange_color); border-radius: 0.5rem; border-right: 0.125rem solid var(--white_color); } button { height: 3.25rem; color: var(--white_color); font-size: 1rem; font-weight: 500; cursor: pointer; margin-top: 0.625rem; background: var(--orange_color); transition: 0.3s ease; padding: 1rem; box-shadow: 0rem 0rem 0.625rem 1rem var(--box_shadow); } button:hover { background: var(--orange_dark_color); } .hide { display: none; } .show { display: block; }
- The CSS file contains styles optimized for the Text To Speech project.
- It defines base styling for fonts, variable definitions for colors, and styling for the container, header, and heading.
- The CSS also includes styling for the Text To Speech section, including input fields, buttons, and the use of CSS variables.
JavaScript
script.js//Text To Speech Variables const textarea = document.querySelector("textarea"), voiceList = document.querySelector("select"), speechBtn = document.getElementById("convert_speech"), clearBtn = document.getElementById("clearBtn"); let synth = speechSynthesis, isSpeaking = true, utterance; voices(); textarea.addEventListener("input", function () { if (textarea.value.length > 0) { // If the textarea has content, add the "show" class to display the button clearBtn.classList.add("show"); clearBtn.classList.remove("hide"); } else { // If the textarea is empty, add the "hide" class to hide the button clearBtn.classList.add("hide"); clearBtn.classList.remove("show"); } }); // Clear Button Function clearBtn.addEventListener("click", e =>{ textarea.value = ""; clearBtn.classList.add("hide"); clearBtn.classList.remove("show"); }); // Select Voice Function function voices(){ for(let voice of synth.getVoices()){ let selected = voice.name === "Google US English" ? "selected" : ""; let option = `<option value="${voice.name}" ${selected}>${voice.name} (${voice.lang})</option>`; voiceList.insertAdjacentHTML("beforeend", option); } } synth.addEventListener("voiceschanged", voices); // Text To Speech Function function textToSpeech(text){ utterance = new SpeechSynthesisUtterance(text); for(let voice of synth.getVoices()){ if(voice.name === voiceList.value){ utterance.voice = voice; } } synth.speak(utterance); } // Speech Button Function speechBtn.addEventListener("click", e =>{ e.preventDefault(); if(textarea.value !== ""){ if(!synth.speaking){ textToSpeech(textarea.value); } if(textarea.value.length > 20){ setInterval(()=>{ if(!synth.speaking && !isSpeaking){ isSpeaking = true; speechBtn.innerText = "Convert To Speech"; }else{ } }, 500); if(isSpeaking){ synth.resume(); isSpeaking = false; speechBtn.innerText = "Pause"; }else{ synth.pause(); isSpeaking = true; speechBtn.innerText = "Resume"; } }else{ speechBtn.innerText = "Convert To Speech"; } } });
- The JavaScript file contains the logic for the Text To Speech functionality.
- It defines variables to access HTML elements like the textarea, voice selection, and buttons.
- The script initializes the speech synthesis and dynamically loads available voices.
- It handles input events, including clearing text, selecting voices, and controlling speech playback based on user interactions.
This web application allows users to enter text and convert it into speech using the browser’s Text To Speech capabilities. The code structure and styling are optimized for user-friendly interaction. It provides options to select voices, control playback, and clear text input for a complete TTS experience. The code is well-commented for clarity and ease of understanding.
YouTube Video
Download Source Code
Don’t forget to share this post!
Click Here : To Show Your Support!