This code represents a simple image gallery web application. Users can view images in a gallery by rotating them in a 3D space. The code includes an HTML file, a CSS file, and a JavaScript file. Below is an explanation of each part:
Folder Structure
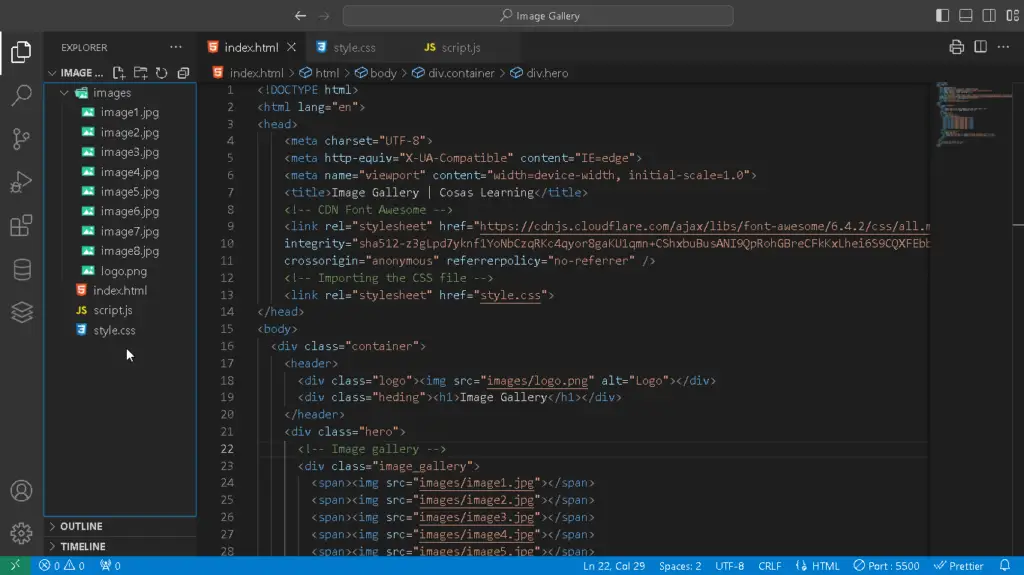
Resources
Prerequisite Sites
Images
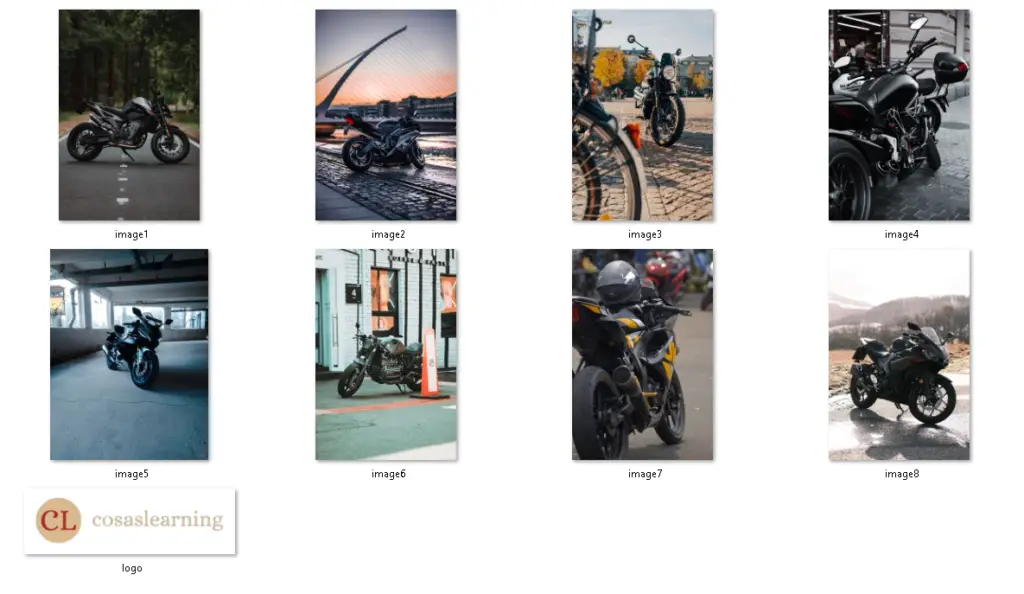
Codes
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Gallery | Cosas Learning</title>
<!-- CDN Font Awesome -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.2/css/all.min.css"
integrity="sha512-z3gLpd7yknf1YoNbCzqRKc4qyor8gaKU1qmn+CShxbuBusANI9QpRohGBreCFkKxLhei6S9CQXFEbbKuqLg0DA=="
crossorigin="anonymous" referrerpolicy="no-referrer" />
<!-- Importing the CSS file -->
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<header>
<div class="logo"><img src="images/logo.png" alt="Logo"></div>
<div class="heding"><h1>Image Gallery</h1></div>
</header>
<div class="hero">
<!-- Image gallery -->
<div class="image_gallery">
<span><img src="images/image1.jpg"></span>
<span><img src="images/image2.jpg"></span>
<span><img src="images/image3.jpg"></span>
<span><img src="images/image4.jpg"></span>
<span><img src="images/image5.jpg"></span>
<span><img src="images/image6.jpg"></span>
<span><img src="images/image7.jpg"></span>
<span><img src="images/image8.jpg"></span>
</div>
<!-- Navigation buttons -->
<div class="img_btns">
<div class="btn prev_btn"><i class="fa-solid fa-angle-left"></i></div>
<div class="btn next_btn"><i class="fa-solid fa-angle-right"></i></div>
</div>
</div>
</div>
<!-- Importing the JavaScript file -->
<script src="script.js"></script>
</body>
</html>
- The HTML file defines the structure of the web page, including the use of Font Awesome icons for arrows and references to external CSS and JavaScript files.
- It contains a container with a header, logo, and the main content section.
- The main content section includes an image gallery with multiple images enclosed in
span
elements. These images can be rotated in a 3D space. - Additionally, there are buttons for navigating the gallery.
CSS
/* CSS styles optimized for the project */
/* Importing Google Font */
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600&display=swap');
/* Base styling */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
/* Variable definitions */
:root {
/* Colors */
--white_color: rgb(255, 255, 255);
--orange_color: rgb(255, 165, 89);
--orange_dark_color: rgb(255, 96, 0);
--black_color: rgb(69, 69, 69);
--background_color: linear-gradient(to top left, #b156d9, #213780);
}
/* Container styling */
.container {
width: 100%;
min-height: 100vh;
background-image: var(--background_color);
padding-bottom: 1rem;
transform-style: preserve-3d;
}
/* Header styling */
header {
padding: 2rem 0;
margin: 0 3rem;
display: flex;
align-items: center;
justify-content: space-between;
}
/* Logo styling */
.logo {
width: 20rem;
height: 6rem;
}
.logo img {
width: 100%;
height: 100%;
}
/* Heading styling */
h1 {
color: var(--white_color);
text-align: center;
padding: 1rem;
border-bottom: 0.2rem var(--orange_color) solid;
text-transform: uppercase;
}
/* ... (CSS rules for the hero section) ... */
.hero {
display: flex;
align-items: center;
justify-content: center;
margin-top: 7rem;
}
/* Image Gallery */
.hero .image_gallery {
position: relative;
width: 15rem;
height: 12.5rem;
transform-style: preserve-3d;
transition: 1.5s;
transform: perspective(62.5rem) rotateY(0deg);
}
.image_gallery span {
position: absolute;
top: 0;
left: 0;
transform-origin: center;
transform-style: preserve-3d;
width: 100%;
height: 100%;
transform: rotateY(0) translateZ(18.75rem);
-webkit-box-reflect: below 0px linear-gradient(transparent, transparent, var(--black_color));
}
.image_gallery span img {
user-select: none;
height: 100%;
width: 100%;
position: absolute;
top: 0;
left: 0;
object-fit: cover;
}
/* ... (CSS rules for the navigation buttons and icons) ... */
.img_btns {
position: absolute;
bottom: 40%;
display: flex;
gap: 56.25rem;
}
.img_btns .btn {
position: relative;
width: 3.75rem;
height: 3.75rem;
border: 0.125rem solid var(--orange_color);
border-radius: 50%;
display: flex;
align-items: center;
justify-content: center;
cursor: pointer;
}
.img_btns .btn:active {
background: var(--white_color);
}
.img_btns .btn:hover {
border: 0.125rem solid var(--orange_dark_color);
}
.img_btns .btn i {
font-size: 2.5rem;
color: var(--orange_color);
}
.img_btns .btn:hover i{
color: var(--orange_dark_color);
}
- The CSS file defines the styling for various elements in the HTML, such as the container, header, logo, hero section, and navigation buttons.
- It uses CSS variables for colors, and it sets up styles to create a visually appealing gallery.
JavaScript
// Image Gallery JS Variables
let image_gallery = document.querySelector('.image_gallery'),
images = image_gallery.querySelectorAll('span'),
prev_btn = document.querySelector('.prev_btn'),
next_btn = document.querySelector('.next_btn');
let deg = 0;
// Creating a 3D Gallery
images.forEach((image, index) => {
const angle = index * 45; // Calculate the rotation angle
image.style.transform = `rotateY(${angle}deg) translateZ(18.75rem)`;
});
// Previous Button
prev_btn.addEventListener('click', function(){
deg += 45;
image_gallery.style.transform = `perspective(62.5rem) rotateY(${deg}deg)`;
})
// Next Button
next_btn.addEventListener('click', function(){
deg -= 45;
image_gallery.style.transform = `perspective(62.5rem) rotateY(${deg}deg)`;
})
- The JavaScript file is responsible for the functionality of the image gallery.
- It selects elements from the HTML using
document.querySelector()
anddocument.querySelectorAll()
. - It sets up a degree variable (
deg
) to track the rotation of the gallery. - It calculates the rotation angle for each image and applies it to create a 3D gallery.
- It adds event listeners to the previous and next buttons, allowing users to navigate the gallery by changing the rotation angle (
deg
).
This code creates a visually appealing 3D image gallery with rotating images and navigation buttons. Users can interact with the gallery to view the images in a unique way. The CSS and JavaScript work together to achieve this effect. You can further customize this gallery by adding more images or modifying the styles.
YouTube Video
Download Source Code
Don’t forget to share this post!
Click Here : To Show Your Support! 😍