Folder Structure
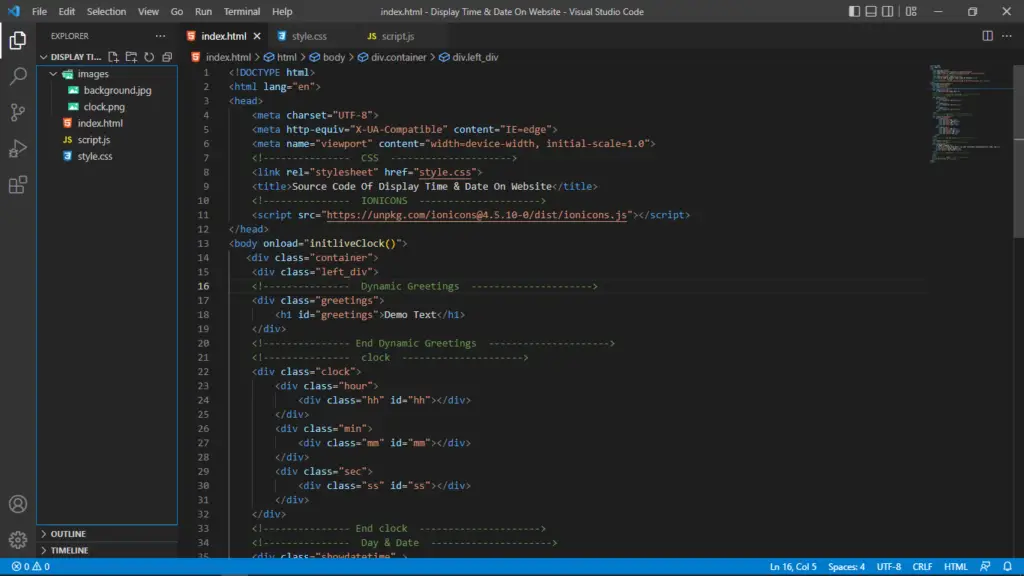
Resources
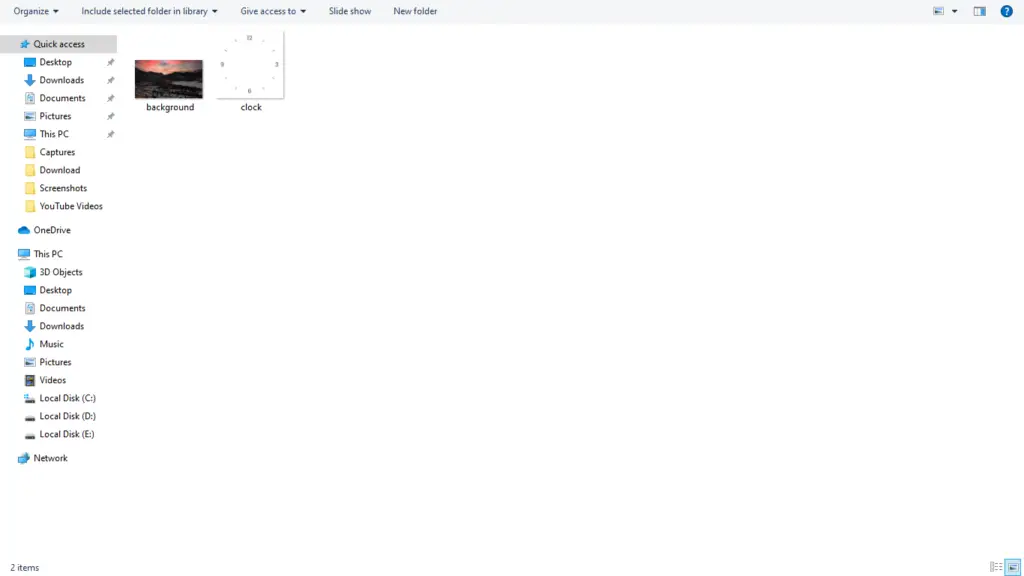
Codes
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!--------------- CSS --------------------->
<link rel="stylesheet" href="style.css">
<title>Source Code Of Display Time & Date On Website</title>
<!--------------- IONICONS --------------------->
<script src="https://unpkg.com/[email protected]/dist/ionicons.js"></script>
</head>
<body onload="initliveClock()">
<div class="container">
<div class="left_div">
<!--------------- Dynamic Greetings --------------------->
<div class="greetings">
<h1 id="greetings">Demo Text</h1>
</div>
<!--------------- End Dynamic Greetings --------------------->
<!--------------- clock --------------------->
<div class="clock">
<div class="hour">
<div class="hh" id="hh"></div>
</div>
<div class="min">
<div class="mm" id="mm"></div>
</div>
<div class="sec">
<div class="ss" id="ss"></div>
</div>
</div>
<!--------------- End clock --------------------->
<!--------------- Day & Date --------------------->
<div class="showdatetime" >
<div class="date">
<span id="day">Day</span>,
<span id="month">Month</span>
<span id="date">00</span>,
<span id="year">Year</span>
</div>
<div class="time">
<span id="hour">00</span>:
<span id="minutes">00</span>:
<span id="seconds">00</span>
<span id="period">AM</span>
</div>
</div>
<!--------------- End Day & Date --------------------->
</div>
<div class="right_div">
<!--------------- Quote --------------------->
<div class="quote">
<h2>TODAY'S QUOTES</h2>
<p id="quote">Lorem ipsum dolor, sit amet consectetur adipisicing elit. Unde, rem.</p>
<h3 id="author">Lorem, ipsum.</h3>
<button id="btn">Get Quote</button>
</div>
<!--------------- End Quote --------------------->
</div>
</div>
<!--------------- SCRIPT --------------------->
<script src="script.js"></script>
</body>
</html>
CSS
/*----------------- GOOGLE FONTS -----------------*/
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;500;600&display=swap');
/*----------------- VARIABLES -----------------*/
:root {
/* Colors */
--orange-color: rgb(246, 99, 53);
--text-color: rgb(96, 97, 99);
--secondary-color: rgb(237, 242, 252);
--white-color: rgb(255, 255, 255);
--para-color:rgb(96, 97, 99);
--dark-color:rgb(27, 31, 41);
--background-color: linear-gradient(rgba(0,0,0,0.5),rgba(0,0,0,0.5))
}
html{
font-size: 10px;
/* Now 1rem = 10px */
}
* {
box-sizing: border-box;
padding: 0;
margin: 0;
font-family: 'Poppins', sans-serif;
}
body {
background: url(images/background.jpg);
background-position: center;
background-size: cover;
width: 100%;
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
}
.container {
width: 90%;
height: 90vh;
background: var(--background-color);
display: flex;
align-items: center;
justify-content: space-around;
}
.left_div {
height: 100%;
width: 60%;
display: flex;
align-items: center;
flex-direction: column;
justify-content: space-around;
}
.right_div{
height: 100%;
width: 40%;
display: flex;
align-items: center;
justify-content: flex-start;
}
/* --------------- clock --------------------- */
.clock {
width: 25rem;
height: 25rem;
display: flex;
justify-content: center;
align-items: center;
background:url(images/clock.png);
background-size: cover;
background-position: center;
border: 0.4rem solid var(--secondary-color);
border-radius: 50%;
box-shadow: 0 -1.5rem 1.5rem rgba(255, 255, 255, 0.05),
inset 0 -1.5rem 1.5rem rgba(255, 255, 255, 0.05),
0 1.5rem 1.5rem rgba(0, 0, 0, 0.03),
inset 0 1.5rem 1.5rem rgba(0, 0, 0, 0.03);
}
.clock::before {
content: '';
position: absolute;
width: 1rem;
height: 1rem;
background: var(--white-color);
border-radius: 50%;
z-index: 1000;
}
.clock .hour, .clock .min, .clock .sec{
position: absolute;
}
.clock .hour, .hh {
width: 10rem;
height: 10rem;
}
.clock .min, .mm {
width: 12rem;
height: 12rem;
}
.clock .sec, .ss {
width: 16rem;
height: 16rem;
}
.hh, .mm, .ss {
display: flex;
justify-content: center;
position: absolute;
border-radius: 50%;
}
.hh:before {
content: '';
position: absolute;
width: 0.8rem;
height: 5rem;
background: var(--orange-color);
z-index: 10;
border-radius: 0.6rem 0.6rem 0 0;
}
.mm:before {
content: '';
position: absolute;
width: 0.4rem;
height: 6rem;
background:var(--white-color);
z-index: 11;
border-radius: 0.6rem 0.6rem 0 0;
}.ss:before {
content: '';
position: absolute;
width: 0.2rem;
height: 10rem;
background: var(--secondary-color);
z-index: 12;
border-radius: 0.6rem 0.6rem 0 0;
}
/* --------------- End clock --------------------- */
/* --------------- Dynamic Greetings --------------------- */
.greetings {
font-size: 1.5rem;
text-align: center;
color: var(--white-color);
text-transform: uppercase;
font-weight: 400;
letter-spacing: 0.1rem;
word-spacing: 0.4rem;
}
/* --------------- End Dynamic Greetings --------------------- */
/* --------------- Date Time --------------------- */
.showdatetime {
color:var(--white-color);
width: 40rem;
padding: 1.5rem 1rem;
}
.date {
font-size: 2rem;
font-weight: 600;
text-align: center;
letter-spacing: 0.3rem;
}
.time {
font-size: 6rem;
display: flex;
justify-content: center;
align-items: center;
}
.time span:not(:last-child){
position: relative;
margin: 0 0.6rem;
font-weight: 600;
text-align: center;
letter-spacing: 0.3rem;
}
.time span:last-child{
font-size: 3rem;
font-weight: 600;
text-transform: uppercase;
margin-top: 1rem;
padding: 0 0.5rem;
}
/* --------------- End Date Time --------------------- */
/* --------------- Quote --------------------- */
.quote {
height: 60%;
width: 80%;
display: flex;
align-items: center;
flex-direction: column;
justify-content: center;
}
.quote h2 {
color: var(--white-color);
text-align: center;
font-size: 3rem;
margin-bottom: 5rem;
}
.quote p {
color: var(--white-color);
text-align: center;
font-size: 1.8rem;
line-height: 2;
letter-spacing: 0.1rem;
}
.quote h3 {
color: var(--orange-color);
margin: 2rem 0 6rem 0;
letter-spacing: 0.2rem;
font-size: 1.8rem;
font-weight: 600;
text-transform: capitalize;
}
.quote button {
background-color: var(--white-color);
border: none;
padding: 1rem 3.5rem;
border-radius: 0.5rem;
font-size: 1.8rem;
letter-spacing: 0.3rem;
font-weight: 600;
color: var(--orange-color);
cursor:pointer;
transition: 0.5s;
}
.quote button:hover {
background-color: var(--orange-color);
color: var(--white-color);
}
/* --------------- End Quote --------------------- */
JavaScript
/* --------------- Dynamic Greetings --------------------- */
let greetings = document.querySelector("#greetings");
let liveTime = new Date().getHours();
let greeting = liveTime >= 5 && liveTime < 12 ? "Good Morning!" :
liveTime >= 12 && liveTime < 18 ? "Good Afternoon!" :
liveTime >= 18 && liveTime < 21 ? "Good Evening!" : "Good Night!";
greetings.innerHTML = greeting;
/* --------------- End Dynamic Greetings --------------------- */
/* --------------- clock --------------------- */
const deg = 6;
const ho = document.querySelector('#hh');
const mi = document.querySelector('#mm');
const se = document.querySelector('#ss');
setInterval(() => {
let day = new Date();
let hh = day.getHours() * 30;
let mm = day.getMinutes() * deg;
let ss = day.getSeconds() * deg;
ho.style.transform = `rotateZ(${(hh)+(mm/12)}deg)`;
mi.style.transform = `rotateZ(${mm}deg)`;
se.style.transform = `rotateZ(${ss}deg)`;
})
/* --------------- End clock --------------------- */
/* --------------- Date Time --------------------- */
function liveClock(){
var liveDate = new Date();
var showDay = liveDate.getDay(),
showMonth = liveDate.getMonth(),
showDate = liveDate.getDate(),
showYear = liveDate.getFullYear(),
showHours = liveDate.getHours(),
showMinutes = liveDate.getMinutes(),
showSeconds = liveDate.getSeconds(),
showPeriod = "AM";
if(showHours == 0){
showHours = 12;
}
if(showHours > 12){
showHours = showHours - 12;
showPeriod = "PM";
}
Number.prototype.pad = function(digits){
for(var n = this.toString(); n.length < digits; n = 0 + n);
return n;
}
var months = ["January", "February", "March", "April", "May", "June", "July",
"Augest", "September", "October", "November", "December"];
var week = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"];
var idNames = ["day", "month", "date", "year", "hour", "minutes",
"seconds", "period"];
var valueNames = [week[showDay], months[showMonth], showDate.pad(2), showYear, showHours.pad(2), showMinutes.pad(2),
showSeconds.pad(2), showPeriod];
for(var i = 0; i < idNames.length; i++)
document.getElementById(idNames[i]).firstChild.nodeValue = valueNames[i];
}
function initliveClock(){
liveClock();
window.setInterval("liveClock()", 1);
}
/* --------------- End Date Time --------------------- */
/* --------------- Quote --------------------- */
let quote = document.getElementById("quote");
let author = document.getElementById("author");
let btn = document.getElementById("btn");
const url = "https://api.quotable.io/random";
let getQuote = () => {
fetch(url)
.then((data) => data.json())
.then((item) => {
quote.innerText = item.content;
author.innerText = item.author;
});
};
window.addEventListener("load", getQuote);
btn.addEventListener("click", getQuote);
window.setInterval("getQuote()", 20000);
/* --------------- End Quote --------------------- */
YouTube Video
Download Source Code
Don’t forget to share this post!
Click Here : To Show Your Support! 😍