This project is a web application that creates an interactive card slider, allowing users to browse through a collection of cards with information about various individuals. The project incorporates HTML, CSS, and JavaScript to achieve this card slider functionality. We have provided an optimized version of the code with comments to explain each step.
Folder Structure
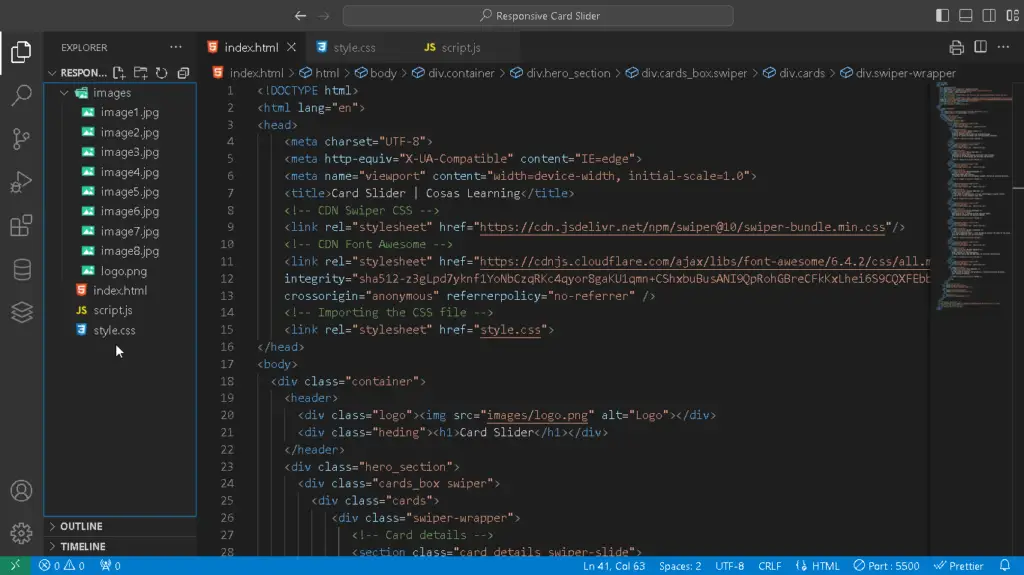
Resources
Prerequisite Sites
Images
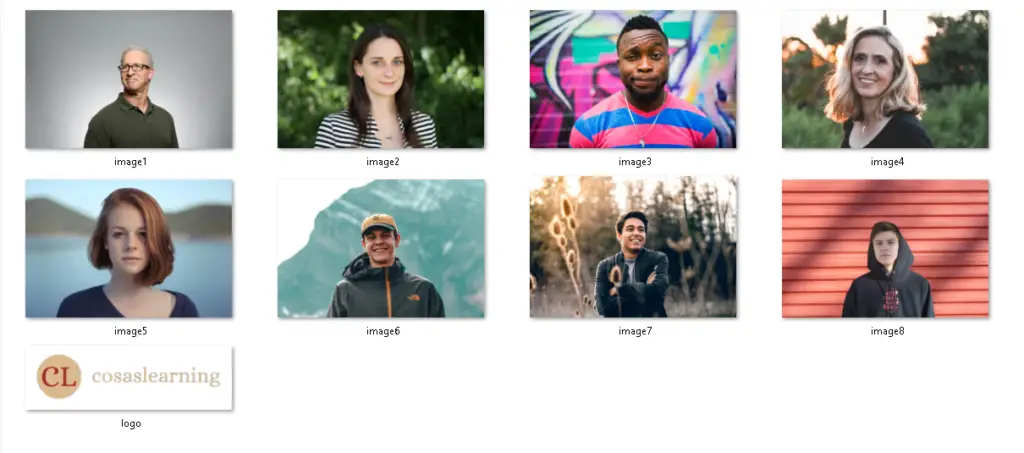
Codes
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Card Slider | Cosas Learning</title>
<!-- CDN Swiper CSS -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/swiper@10/swiper-bundle.min.css"/>
<!-- CDN Font Awesome -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.2/css/all.min.css"
integrity="sha512-z3gLpd7yknf1YoNbCzqRKc4qyor8gaKU1qmn+CShxbuBusANI9QpRohGBreCFkKxLhei6S9CQXFEbbKuqLg0DA=="
crossorigin="anonymous" referrerpolicy="no-referrer" />
<!-- Importing the CSS file -->
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<header>
<div class="logo"><img src="images/logo.png" alt="Logo"></div>
<div class="heding"><h1>Card Slider</h1></div>
</header>
<div class="hero_section">
<div class="cards_box swiper">
<div class="cards">
<div class="swiper-wrapper">
<!-- Card details -->
<section class="card_details swiper-slide">
<div class="card_img_box">
<img src="images/image1.jpg" class="card_img">
</div>
<div class="card_data">
<h3 class="card_name">Samuel Techton</h3>
<p class="card_description">
Software engineer with a knack for problem-solving,
I thrive on coding challenges and crafting elegant solutions.
</p>
<a href="#" class="card_button">Follow</a>
</div>
</section>
<!-- Repeat similar sections for other cards -->
<section class="card_details swiper-slide">
<div class="card_img_box">
<img src="images/image2.jpg" class="card_img">
</div>
<div class="card_data">
<h3 class="card_name">Emily Codecraft</h3>
<p class="card_description">
Dedicated to making the digital world more user-friendly,
I specialize in UI/UX design and interface optimization.
</p>
<a href="#" class="card_button">Follow</a>
</div>
</section>
<section class="card_details swiper-slide">
<div class="card_img_box">
<img src="images/image3.jpg" class="card_img">
</div>
<div class="card_data">
<h3 class="card_name">David Datawizard</h3>
<p class="card_description">
Passionate about data analysis,
I transform raw data into actionable insights that drive informed decisions.
</p>
<a href="#" class="card_button">Follow</a>
</div>
</section>
<section class="card_details swiper-slide">
<div class="card_img_box">
<img src="images/image4.jpg" class="card_img">
</div>
<div class="card_data">
<h3 class="card_name">Jessica Cyberstar</h3>
<p class="card_description">
In the realm of cybersecurity, I'm your shield against digital threats.
I ensure your online presence stays safe.
</p>
<a href="#" class="card_button">Follow</a>
</div>
</section>
<section class="card_details swiper-slide">
<div class="card_img_box">
<img src="images/image5.jpg" class="card_img">
</div>
<div class="card_data">
<h3 class="card_name">Olivia AIwhisper</h3>
<p class="card_description">
Fascinated by AI, I develop machine learning models
that enhance automation and decision-making.
</p>
<a href="#" class="card_button">Follow</a>
</div>
</section>
<section class="card_details swiper-slide">
<div class="card_img_box">
<img src="images/image6.jpg" class="card_img">
</div>
<div class="card_data">
<h3 class="card_name">Rachel Cloudmaven</h3>
<p class="card_description">
As a cloud computing expert, I help businesses harness the power of the cloud,
ensuring scalability, and cost-efficiency.
</p>
<a href="#" class="card_button">Follow</a>
</div>
</section>
<section class="card_details swiper-slide">
<div class="card_img_box">
<img src="images/image7.jpg" class="card_img">
</div>
<div class="card_data">
<h3 class="card_name">Michael Appsmith</h3>
<p class="card_description">
With a passion for app development,
I bring your mobile and web app ideas to life with functionality.
</p>
<a href="#" class="card_button">Follow</a>
</div>
</section>
<section class="card_details swiper-slide">
<div class="card_img_box">
<img src="images/image8.jpg" class="card_img">
</div>
<div class="card_data">
<h3 class="card_name">Alex Robotech</h3>
<p class="card_description">
In the world of robotics and automation, I create innovative solutions
that improve efficiency.
</p>
<a href="#" class="card_button">Follow</a>
</div>
</section>
</div>
</div>
<!-- Navigation buttons -->
<div class="swiper-button-next">
<i class="fa-solid fa-angle-right"></i>
</div>
<div class="swiper-button-prev">
<i class="fa-solid fa-angle-left"></i>
</div>
<!-- Pagination -->
<div class="swiper-pagination"></div>
</div>
</div>
</div>
<!-- CDN Swiper JavaScript -->
<script src="https://cdn.jsdelivr.net/npm/swiper@10/swiper-bundle.min.js"></script>
<!-- Importing the JavaScript file -->
<script src="script.js"></script>
</body>
</html>
- The HTML file sets up the structure of the web page, including references to external CSS and JavaScript files, as well as external resources like the Swiper CSS and Font Awesome for icons.
- It defines a container with a header, logo, heading, and a hero_section Div.
- Inside the card slider section, individual cards are created using the Swiper structure, and each card contains details about an individual, including their image, name, description, and a “Follow” button.
CSS
/* CSS styles optimized for the project */
/* Importing Google Font */
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600&display=swap');
/* Base styling */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
/* Variable definitions */
:root {
/* Colors */
--white_color: rgb(255, 255, 255);
--orange_color: rgb(255, 165, 89);
--orange_dark_color: rgb(255, 96, 0);
--black_color: rgb(69, 69, 69);
--background_color: linear-gradient(to top left, #b156d9, #213780);
--font_color : rgb(255, 230, 199);
}
/* Container styling */
.container {
width: 100%;
min-height: 100vh;
background-image: var(--background_color);
padding-bottom: 1rem;
}
/* Header styling */
header {
padding: 2rem 0;
margin: 0 3rem;
display: flex;
align-items: center;
justify-content: space-between;
}
/* Logo styling */
.logo {
width: 20rem;
height: 6rem;
}
.logo img {
width: 100%;
height: 100%;
}
/* Heading styling */
h1 {
color: var(--white_color);
text-align: center;
padding: 1rem;
border-bottom: 0.2rem var(--orange_color) solid;
text-transform: uppercase;
}
/* Card styling */
.cards_box {
margin: 0 5rem;
padding: 3.5rem 5rem;
}
a {
text-decoration: none;
}
img {
display: block;
max-width: 100%;
height: auto;
}
.cards {
max-width: 1120px;
border-radius: 1.25rem;
overflow: hidden;
}
.card_details {
width: 300px;
border-radius: 1.25rem;
overflow: hidden;
}
.card_img_box {
position: relative;
background-color: var(--orange_color);
padding-top: .75rem;
margin-bottom: -.75rem;
}
.card_data {
background: var(--black_color);
padding: 1.5rem 2rem;
border-radius: 1rem;
text-align: center;
position: relative;
z-index: 10;
}
.card_img {
width: 300px;
border-radius: 1rem;
margin: 0 auto;
position: relative;
z-index: 5;
}
.card_name {
font-size: 1rem;
color: var(--font_color);
margin-bottom: .75rem;
}
.card_description {
font-weight: 500;
margin-bottom: 1.75rem;
color: var(--white_color);
}
.card_button {
display: inline-block;
background-color: var(--orange_color);
padding: .75rem 1.5rem;
border-radius: .25rem;
color: var(--white_color);
font-weight: 600;
}
/* Swiper class */
.swiper-button-prev:after,
.swiper-button-next:after {
content: "";
}
.swiper-button-prev, .swiper-button-next {
width: initial;
height: initial;
font-size: 3rem;
color: var(--orange_color);
}
.swiper-button-prev:hover,
.swiper-button-next:hover {
color: var(--orange_dark_color);
}
.swiper-button-prev {
left: 0;
}
.swiper-button-next {
right: 0;
}
.swiper-pagination-bullet {
background-color:var(--orange_color);
opacity: 1;
}
.swiper-pagination-bullet-active,
.card_button:hover {
background-color: var(--orange_dark_color);
}
- The CSS file defines styles optimized for the project, including font imports, base styling, and variables for colors and fonts.
- It styles the container, header, logo, heading, card elements, and swiper class ensuring a visually appealing layout for the card slider.
JavaScript
// Swiper JavaScript Code
let swiperCards = new Swiper(".cards", {
loop: true,
spaceBetween: 32,
grabCursor: true,
pagination: {
el: ".swiper-pagination",
clickable: true,
dynamicBullets: true,
},
navigation: {
nextEl: ".swiper-button-next",
prevEl: ".swiper-button-prev",
},
breakpoints:{
968: {
slidesPerView: 3,
},
},
});
- The JavaScript file initializes Swiper, a popular slider library, to create the card slider functionality.
- It sets options like loop, space between cards, and navigation controls.
- The code also includes responsive breakpoints for the slider’s behavior on different screen sizes.
This web application provides users with an interactive card slider that allows them to browse through individual cards with detailed information. It leverages external libraries like Swiper and Font Awesome for enhanced functionality and icons. The code structure and styling have been optimized and well-commented for clarity and a visually appealing user experience. You can customize it further.
YouTube Video
Download Source Code
Don’t forget to share this post!
Click Here : To Show Your Support! 😍